基于LPC1114的学习型红外遥控器
#include
#include
//---------------------------------------------------------
void sysint(void);
//---------------------------------------------------------
main(void)
{
uint32_t i;
/* Enable AHB clock to the GPIO domain. */
LPC_SYSCON->SYSAHBCLKCTRL |= (1<<6);
/* Set up NVIC when I/O pins are configured as external interrupts. */
NVIC_EnableIRQ(EINT0_IRQn);
NVIC_EnableIRQ(EINT1_IRQn);
NVIC_EnableIRQ(EINT2_IRQn);
NVIC_EnableIRQ(EINT3_IRQn);
LPC_GPIO0->DIR=0xFF;
while(1)
{
LPC_GPIO0->DATA=~LPC_GPIO0->DATA;
i=300000;
while(i--);
}
}
//---------------------------------------------------------
void sysint (void)
{
// uint32_t i;
#ifdef __DEBUG_RAM
LPC_SYSCON->SYSMEMREMAP = 0x1; /* remap to internal RAM */
#else
#ifdef __DEBUG_FLASH
LPC_SYSCON->SYSMEMREMAP = 0x2; /* remap to internal flash */
#endif
#endif
#if (CLOCK_SETUP) /* Clock Setup */
/* bit 0 default is crystal bypass,
bit1 0=0~20Mhz crystal input, 1=15~50Mhz crystal input. */
LPC_SYSCON->SYSOSCCTRL = 0x00;
/* main system OSC run is cleared, bit 5 in PDRUNCFG register */
LPC_SYSCON->PDRUNCFG &= ~(0x1<<5);
/* Wait 200us for OSC to be stablized, no status
indication, dummy wait. */
for ( i = 0; i < 0x100; i++ );
#if (MAIN_PLL_SETUP)
Main_PLL_Setup();
#endif
#endif /* endif CLOCK_SETUP */
/* System clock to the IOCON needs to be enabled or
most of the I/O related peripherals won't work. */
LPC_SYSCON->SYSAHBCLKCTRL |= (1<<16);
return;
}
心情:利用了2天的业余时间,终于LED灯闪亮了,感觉LPC1114的功能真的很强大,比一般的51单片机复杂的多。以后要多努力学习了。
不知不觉已经11点多了。在喝一瓶,洗洗睡了。
GPIO"库"函数中文说明
NXP的LPC11XX功能很强大。"库"函数也非常多,功能也很强大,充分理解"库"函数能帮助我们快速学习LPC11XX,下面是英文和中文"库"函数的翻译,希望能帮助大家快速学习LPC11XX,以后我会抽出时间,翻译其他功能的"库"函数。
/*****************************************************************************
* gpio.c: GPIO C file for NXP LPC11xx Family Microprocessors
*
* Copyright(C) 2008, NXP Semiconductor
* All rights reserved.
*
* History
* 2008.07.20 ver 1.00 Prelimnary version, first Release
*
*****************************************************************************/
#include "LPC11xx.h" /* LPC11xx Peripheral Registers */
#include "gpio.h"
/* Shadow registers used to prevent chance of read-modify-write errors */
/* Ultra-conservative approach... */
volatile uint32_t GPIOShadowPort0;
volatile uint32_t GPIOShadowPort1;
volatile uint32_t GPIOShadowPort2;
volatile uint32_t GPIOShadowPort3;
volatile uint32_t gpio0_counter = 0;
volatile uint32_t gpio1_counter = 0;
volatile uint32_t gpio2_counter = 0;
volatile uint32_t gpio3_counter = 0;
volatile uint32_t p0_1_counter = 0;
volatile uint32_t p1_1_counter = 0;
volatile uint32_t p2_1_counter = 0;
volatile uint32_t p3_1_counter = 0;
/*****************************************************************************
** Function name: PIOINT0_IRQHandler
**
** Descriptions: Use one GPIO pin(port0 pin1) as interrupt source
**
** parameters: None
** Returned value: None
**
*****************************************************************************/
void PIOINT0_IRQHandler(void)
{
uint32_t regVal;
gpio0_counter++;
regVal = GPIOIntStatus( PORT0, 1 );
if ( regVal )
{
p0_1_counter++;
GPIOIntClear( PORT0, 1 );
}
return;
}
/*****************************************************************************
** Function name: PIOINT1_IRQHandler
**
** Descriptions: Use one GPIO pin(port1 pin1) as interrupt source
**
** parameters: None
** Returned value: None
**
*****************************************************************************/
void PIOINT1_IRQHandler(void)
{
uint32_t regVal;
gpio1_counter++;
regVal = GPIOIntStatus( PORT1, 1 );
if ( regVal )
{
p1_1_counter++;
GPIOIntClear( PORT1, 1 );
}
return;
}
/*****************************************************************************
** Function name: PIOINT2_IRQHandler
**
** Descriptions: Use one GPIO pin(port2 pin1) as interrupt source
**
** parameters: None
** Returned value: None
**
*****************************************************************************/
void PIOINT2_IRQHandler(void)
{
uint32_t regVal;
gpio2_counter++;
regVal = GPIOIntStatus( PORT2, 1 );
if ( regVal )
{
p2_1_counter++;
GPIOIntClear( PORT2, 1 );
}
return;
}
/*****************************************************************************
** Function name: PIOINT3_IRQHandler
**
** Descriptions: Use one GPIO pin(port3 pin1) as interrupt source
**
** parameters: None
** Returned value: None
**
*****************************************************************************/
void PIOINT3_IRQHandler(void)
{
uint32_t regVal;
gpio3_counter++;
regVal = GPIOIntStatus( PORT3, 1 );
if ( regVal )
{
p3_1_counter++;
GPIOIntClear( PORT3, 1 );
}
return;
}
/*****************************************************************************
** Function name: GPIOInit
**
** Descriptions: Initialize GPIO, install the
** GPIO interrupt handler
**
** parameters: None
** Returned value: true or false, return false if the VIC table
** is full and GPIO interrupt handler can be
** installed.
**
*****************************************************************************/
void GPIOInit( void )
{
/* Enable AHB clock to the GPIO domain. */
LPC_SYSCON->SYSAHBCLKCTRL |= (1<<6);
#ifdef __JTAG_DISABLED
LPC_IOCON->JTAG_TDO_PIO1_1 &= ~0x07;
LPC_IOCON->JTAG_TDO_PIO1_1 |= 0x01;
#endif
/* Set up NVIC when I/O pins are configured as external interrupts. */
NVIC_EnableIRQ(EINT0_IRQn);
NVIC_EnableIRQ(EINT1_IRQn);
NVIC_EnableIRQ(EINT2_IRQn);
NVIC_EnableIRQ(EINT3_IRQn);
return;
}
/*****************************************************************************
** Function name: GPIOSetDir
**
** Descriptions: Set the direction in GPIO port
**
** parameters: port num, bit position, direction (1 out, 0 input)
** Returned value: None
**
*****************************************************************************/
void GPIOSetDir( uint32_t portNum, uint32_t bitPosi, uint32_t dir )
{
/* if DIR is OUT(1), but GPIOx_DIR is not set, set DIR
to OUT(1); if DIR is IN(0), but GPIOx_DIR is set, clr
DIR to IN(0). All the other cases are ignored.
On port3(bit 0 through 3 only), no error protection if
bit value is out of range. */
switch ( portNum )
{
case PORT0:
if ( !(LPC_GPIO0->DIR & (0x1< LPC_GPIO0->DIR |= (0x1< else if ( (LPC_GPIO0->DIR & (0x1< LPC_GPIO0->DIR &= ~(0x1< break;
case PORT1:
if ( !(LPC_GPIO1->DIR & (0x1< LPC_GPIO1->DIR |= (0x1< else if ( (LPC_GPIO1->DIR & (0x1< LPC_GPIO1->DIR &= ~(0x1< break;
case PORT2:
if ( !(LPC_GPIO2->DIR & (0x1< LPC_GPIO2->DIR |= (0x1< else if ( (LPC_GPIO2->DIR & (0x1< LPC_GPIO2->DIR &= ~(0x1< break;
case PORT3:
if ( !(LPC_GPIO3->DIR & (0x1< LPC_GPIO3->DIR |= (0x1< else if ( (LPC_GPIO3->DIR & (0x1< LPC_GPIO3->DIR &= ~(0x1< break;
default:
break;
}
return;
}
/*****************************************************************************
** Function name: GPIOSetValue
**
** Descriptions: Set/clear a bitvalue in a specific bit position
** in GPIO portX(X is the port number.)
**
** parameters: port num, bit position, bit value
** Returned value: None
**
*****************************************************************************/
void GPIOSetValue( uint32_t portNum, uint32_t bitPosi, uint32_t bitVal )
{
/* if bitVal is 1, the bitPosi bit is set in the GPIOShadowPortx. Then
* GPIOShadowPortx is written to the I/O port register. */
switch ( portNum )
{
case PORT0:
if(bitVal)
GPIOShadowPort0 |= (1< else
GPIOShadowPort0 &= ~(1<
/* Use of shadow prevents bit operation error if the read value
* (external hardware state) of a pin differs from the I/O latch
* value. A potential side effect is that other GPIO code in this
* project that is not aware of the shadow will have its GPIO
* state overwritten.
*/
LPC_GPIO0->DATA = GPIOShadowPort0;
break;
case PORT1:
if(bitVal)
GPIOShadowPort1 |= (1< else
GPIOShadowPort1 &= ~(1<
LPC_GPIO1->DATA = GPIOShadowPort1;
break;
case PORT2:
if(bitVal)
GPIOShadowPort2 |= (1< else
GPIOShadowPort2 &= ~(1<
LPC_GPIO2->DATA = GPIOShadowPort2;
break;
case PORT3:
if(bitVal)
GPIOShadowPort3 |= (1< else
GPIOShadowPort3 &= ~(1<
LPC_GPIO3->DATA = GPIOShadowPort3;
break;
default:
break;
}
return;
}
/*****************************************************************************
** Function name: GPIOSetInterrupt
**
** Descriptions: Set interrupt sense, event, etc.
** edge or level, 0 is edge, 1 is level
** single or double edge, 0 is single, 1 is double
** active high or low, etc.
**
** parameters: port num, bit position, sense, single/doube, polarity
** Returned value: None
**
*****************************************************************************/
void GPIOSetInterrupt( uint32_t portNum, uint32_t bitPosi, uint32_t sense,
uint32_t single, uint32_t event )
{
switch ( portNum )
{
case PORT0:
if ( sense == 0 )
{
LPC_GPIO0->IS &= ~(0x1< /* single or double only applies when sense is 0(edge trigger). */
if ( single == 0 )
LPC_GPIO0->IBE &= ~(0x1< else
LPC_GPIO0->IBE |= (0x1< }
else
LPC_GPIO0->IS |= (0x1< if ( event == 0 )
LPC_GPIO0->IEV &= ~(0x1< else
LPC_GPIO0->IEV |= (0x1< break;
case PORT1:
if ( sense == 0 )
{
LPC_GPIO1->IS &= ~(0x1< /* single or double only applies when sense is 0(edge trigger). */
if ( single == 0 )
LPC_GPIO1->IBE &= ~(0x1< else
LPC_GPIO1->IBE |= (0x1< }
else
LPC_GPIO1->IS |= (0x1< if ( event == 0 )
LPC_GPIO1->IEV &= ~(0x1< else
LPC_GPIO1->IEV |= (0x1< break;
case PORT2:
if ( sense == 0 )
{
LPC_GPIO2->IS &= ~(0x1< /* single or double only applies when sense is 0(edge trigger). */
if ( single == 0 )
LPC_GPIO2->IBE &= ~(0x1< else
LPC_GPIO2->IBE |= (0x1< }
else
LPC_GPIO2->IS |= (0x1< if ( event == 0 )
LPC_GPIO2->IEV &= ~(0x1< else
LPC_GPIO2->IEV |= (0x1< break;
case PORT3:
if ( sense == 0 )
{
LPC_GPIO3->IS &= ~(0x1< /* single or double only applies when sense is 0(edge trigger). */
if ( single == 0 )
LPC_GPIO3->IBE &= ~(0x1< else
LPC_GPIO3->IBE |= (0x1< }
else
LPC_GPIO3->IS |= (0x1< if ( event == 0 )
LPC_GPIO3->IEV &= ~(0x1< else
LPC_GPIO3->IEV |= (0x1< break;
default:
break;
}
return;
}
/*****************************************************************************
** Function name: GPIOIntEnable
**
** Descriptions: Enable Interrupt Mask for a port pin.
**
** parameters: port num, bit position
** Returned value: None
**
*****************************************************************************/
void GPIOIntEnable( uint32_t portNum, uint32_t bitPosi )
{
switch ( portNum )
{
case PORT0:
LPC_GPIO0->IE |= (0x1< break;
case PORT1:
LPC_GPIO1->IE |= (0x1< break;
case PORT2:
LPC_GPIO2->IE |= (0x1< break;
case PORT3:
LPC_GPIO3->IE |= (0x1< break;
default:
break;
}
return;
}
/*****************************************************************************
** Function name: GPIOIntDisable
**
** Descriptions: Disable Interrupt Mask for a port pin.
**
** parameters: port num, bit position
** Returned value: None
**
*****************************************************************************/
void GPIOIntDisable( uint32_t portNum, uint32_t bitPosi )
{
switch ( portNum )
{
case PORT0:
LPC_GPIO0->IE &= ~(0x1< break;
case PORT1:
LPC_GPIO1->IE &= ~(0x1< break;
case PORT2:
LPC_GPIO2->IE &= ~(0x1< break;
case PORT3:
LPC_GPIO3->IE &= ~(0x1< break;
default:
break;
}
return;
}
/*****************************************************************************
** Function name: GPIOIntStatus
**
** Descriptions: Get Interrupt status for a port pin.
**
** parameters: port num, bit position
** Returned value: None
**
*****************************************************************************/
uint32_t GPIOIntStatus( uint32_t portNum, uint32_t bitPosi )
{
uint32_t regVal = 0;
switch ( portNum )
{
case PORT0:
if ( LPC_GPIO0->MIS & (0x1< regVal = 1;
break;
case PORT1:
if ( LPC_GPIO1->MIS & (0x1< regVal = 1;
break;
case PORT2:
if ( LPC_GPIO2->MIS & (0x1< regVal = 1;
break;
case PORT3:
if ( LPC_GPIO3->MIS & (0x1< regVal = 1;
break;
default:
break;
}
return ( regVal );
}
/*****************************************************************************
** Function name: GPIOIntClear
**
** Descriptions: Clear Interrupt for a port pin.
**
** parameters: port num, bit position
** Returned value: None
**
*****************************************************************************/
void GPIOIntClear( uint32_t portNum, uint32_t bitPosi )
{
switch ( portNum )
{
case PORT0:
LPC_GPIO0->IC |= (0x1< break;
case PORT1:
LPC_GPIO1->IC |= (0x1< break;
case PORT2:
LPC_GPIO2->IC |= (0x1< break;
case PORT3:
LPC_GPIO3->IC |= (0x1< break;
default:
break;
}
return;
}
/******************************************************************************
** End Of File
******************************************************************************/
/*****************************************************************************
** 函数名: GPIOSetDir
** 功能: 设置GPIO口方向
** 参数: 端口号(PORT0-PORT3), 第几位(0-31), 方向 (1 输出, 0 输入)
** 返回值: 无
*****************************************************************************/
/*****************************************************************************
** 函数名: GPIOSetValue
** 功能: 设置端口的值
** 参数: 端口号(PORT0-PORT3), 第几位(0-31), 方向 (1 输出, 0 输入)
** 返回值: 无
*****************************************************************************/
/*****************************************************************************
** 函数名: GPIOSetInterrupt
** 功能: 设置中断
** 参数: 端口号(PORT0-PORT3),
第几位(0-31),
触发方式(边缘触发还是电平触发 0边缘,1电平),
单/双电平触发,(0单电平,1,双电平),
根据触发方式选类型(0上升沿或高电平,1下降沿或低电平)
** 返回值: 无
*****************************************************************************/
/*****************************************************************************
** 函数名: GPIOIntEnable
** 功能: 允许I/O引脚中断
** 参数: 端口号(PORT0-PORT3), 第几位(0-31),
** 返回值: 无
*****************************************************************************/
/*****************************************************************************
** 函数名: GPIOIntDisable
** 功能: 禁止I/O引脚中断
** 参数: 端口号(PORT0-PORT3), 第几位(0-31),
** 返回值: 无
*****************************************************************************/
/*****************************************************************************
** 函数名: GPIOIntStatus
** 功能: 获得引脚中断状态
** 参数: 端口号(PORT0-PORT3), 第几位(0-31),
** 返回值: 0 无中断或中断屏蔽,1 有中断
*****************************************************************************/
/*****************************************************************************
** 函数名: GPIOIntClear
** 功能: 清除边沿检测逻辑
** 参数: 端口号(PORT0-PORT3), 第几位(0-31),
** 返回值: 无
*****************************************************************************/
/*****************************************************************************
** 函数名: GPIOInit
** 功能: GPIO初始化
** 参数: 无
** 返回值: 真或假,(一般不用)。
*****************************************************************************/
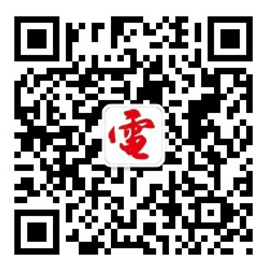
加入微信
获取电子行业最新资讯
搜索微信公众号:EEPW
或用微信扫描左侧二维码